Thank you for the explanation!
I didn’t see the changes in the tilemap.vp 
I’ve easily fixed by getting the world transform from the game object then inverting it.
With the inverse matrix in the shader I can tranform back position in object space. 
But … 
The only tricky thing is passing a matrix4 into the material because tilemap.set_constant only supports vector4, so it requires 4 uniforms.
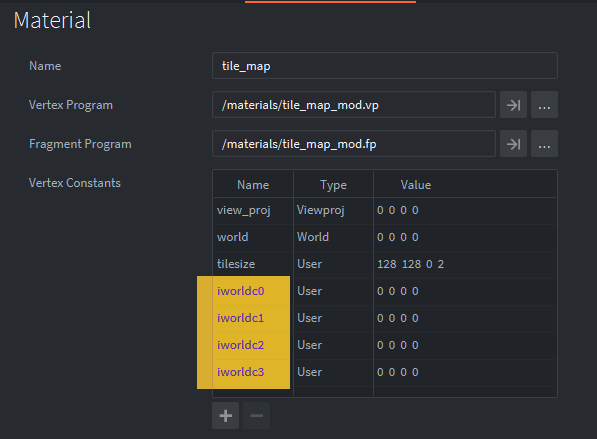
The init function the matrix is splitted …
function init(self)
self.tilemap="#level_animator"
self.half_inteval=self.interval/2
self.elapsed=self.half_inteval-self.speed*2
self.time_pos=-self.half_inteval
local x,y,w,h=tilemap.get_bounds(self.tilemap)
tilemap.set_constant(self.tilemap,"bounds", vmath.vector4(x,y,w,h))
local world=go.get_world_transform()
local iworld=vmath.inv(world)
tilemap.set_constant(self.tilemap,"iworldc0", iworld.c0)
tilemap.set_constant(self.tilemap,"iworldc1", iworld.c1)
tilemap.set_constant(self.tilemap,"iworldc2", iworld.c2)
tilemap.set_constant(self.tilemap,"iworldc3", iworld.c3)
update_tdso(self)
compute(self)
end
And in the shared is recomposed …
uniform highp mat4 view_proj;
uniform lowp vec4 tilesize;
attribute highp vec4 position;
attribute mediump vec2 texcoord0;
varying mediump vec2 var_texcoord0;
varying mediump vec2 tilexy;
uniform mediump vec4 iworldc0;
uniform mediump vec4 iworldc1;
uniform mediump vec4 iworldc2;
uniform mediump vec4 iworldc3;
void main()
{
gl_Position = view_proj * vec4(position.xyz, 1.0);
var_texcoord0 = texcoord0;
mat4 mediump iworld=mat4(iworldc0,iworldc1,iworldc2,iworldc3);
vec4 highp iposition=iworld * vec4(position.xyz, 1.0);
tilexy=vec2(iposition.x/tilesize.x,iposition.y/tilesize.y);
}
And so the next question(s) are:
- Allowing to pass an matrix4 to material?
- Or better, add the inverse world transformation matrix as chooseable option when building a material .
Thanks in advance