For a few of my games I used a module system combined with inheritance. I come from an Object-Oriented Programming background, so it makes the most sense to my mind. I don’t like copy / pasting functions since that makes fixing bugs and adding functionality harder.
It’s worked well for me in the games I’ve made. However, I’ve never tried with 500-1,000 items.
Here’s the approach I used in Rogue Royale Reloaded (50-60 items):
At the high level I had an item module with a name, ID, and some functions common across all items (can spawn as loot, use item, load, save, etc).
From there I created several sub-modules for ammo types, weapons, clothing, etc. I could change or add functions that would be used by all members of that class. Clothing would have a health value, weapons would override the ‘use item’ function to shoot. Etc.
Finally I would create a module for the item that had the description, ID, and any other functions that would be specifically needed by that item.
In the end the structure looked something like this:
- item
– Clothing
— Armor
---- Knight Armor, Suit, Space Suit, etc
— Helmet
— Etc
I did keep a singleton file with all of the items in a table so I quickly grab items by ID.
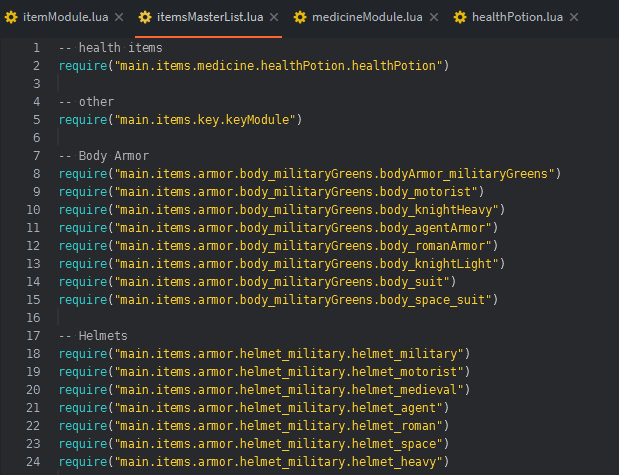
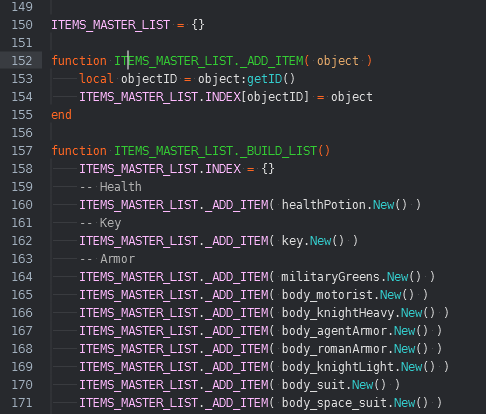
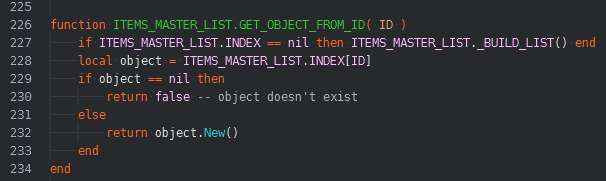
Hope this helps!