Okay. There is something stranger happening here.
After getting some help on the slack channel, i decided to see what would happen if i stopped rotating the text to form a circle and just did the following code.
message is a table with a load of letters.
And, the message gets sent twice, to avoid the problem of not being able to get label metrics in the same frame as setting a label.
elseif message_id == hash("text") then
print("------------ new message: ------------" )
self.accumulateddistance = 0
for k, v in pairs(message) do
local mylabel = ("small object"..k.."#label")
local myobj = ("/textcirclecollection/small object"..k)
local metrics = label.get_text_metrics(mylabel)
self.accumulateddistance = self.accumulateddistance + metrics.width
go.set(myobj, "position.x", (self.accumulateddistance))
end
--then we send the new letters
for k, v in pairs(message) do
local mylabel = ("small object"..k.."#label")
if message[k] == "-"then
label.set_text(mylabel, "-")
go.set(mylabel, "color.w", 0)
else
label.set_text(mylabel, message[k])
go.set(mylabel, "color.w", 1)
end
end
The code appears to work well:
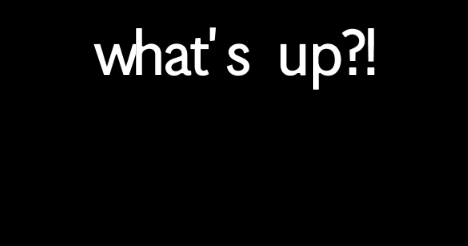
but has some unexpected problems with some letters. It’s weird.
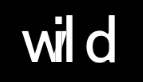
Why is that?