Yes, syntax is like in C++ :
if(){}
or
if(){}else if(){} else{}
To do what you want (pallete modifications) take a default sprite.fp as a base of your new fragment program:
varying mediump vec2 var_texcoord0;
uniform lowp sampler2D texture_sampler;
uniform lowp vec4 tint;
void main()
{
// Pre-multiply alpha since all runtime textures already are
lowp vec4 tint_pm = vec4(tint.xyz * tint.w, tint.w);
gl_FragColor = texture2D(texture_sampler, var_texcoord0.xy) * tint_pm;
}
above, the color of the pixel is taken (from texture2D) and only multiplied by tint_pm and written to gl_FragColor which is an output color.
You want to take a color from texture and not write it to the output, but to a variable, that you can operate on, so make a vector called color_of_pixel and take a color from texture2D:
lowp vec4 color_of_pixel = texture2D(texture_sampler, var_texcoord0.xy);
Then you want to modify the color if it meets your requirement, so here you can write for example:
if (color_of_pixel == base_hair_color) {
pixel_color = my_new_hair;
}
^ This will take the pixels that has the same color as base_hair_color (e.g. black) and change its color to my_new_hair e.g.white;
Don’t forget to define the colors you used in if statement on top of the program or pass it using a shader constant (more advanced).
lowp vec4 base_hair_color = vec4(0.0, 0.0, 0.0, 1.0); // black - i just wrote it on top of program
lowp vec4 my_new_hair = vec4(1.0, 1.0, 1.0, 1.0); // white- i just wrote it on top of program
Write as many if statement as you need using else if
construct
In the end write the color_of_pixel to the output variable called gl_FragColor and eventually multiply it by the tint as in the original sprite fragment program.
The whole program can look like this:
varying mediump vec2 var_texcoord0;
uniform lowp sampler2D texture_sampler;
uniform lowp vec4 tint;
lowp vec4 base_hair_color = vec4(0.0, 0.0, 0.0, 1.0); // black - i just wrote it on top of program
lowp vec4 my_new_hair = vec4(1.0, 1.0, 1.0, 1.0); // white- i just wrote it on top of program
void main()
{
// Pre-multiply alpha since all runtime textures already are
lowp vec4 tint_pm = vec4(tint.xyz * tint.w, tint.w);
lowp vec4 color_of_pixel = texture2D(texture_sampler, var_texcoord0.xy);
if (color_of_pixel == base_hair_color) {
pixel_color = my_new_hair;
}
gl_FragColor = color_of_pixel * tint_pm;
}
Then add such program to a new material and apply this material to a sprite (with black hair
)
Run it and hair should be white 
This is the most simplest version and will work for all black pixels, so I;m using special colors for sprites I want such modifications to have (aka only hero sprite:)
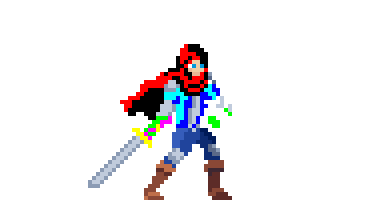
You see here I used a specific color pallete - cloak, gloves, sword and jacket are modifiable and use basic colors (red, green, blue, magenta, cyan, yellow, black, white) so in shader program for that particular sprite there is no option that when I change color for cloak, some other colors will be changed, because there is no other part using pure red nor black color
Tinker a little bit with it and maybe you won’t need to recolour your sprites
just getting RGB values of your current sprites will be enough (I did that for the first version, something like: if (color == vec4(55/255, 60/255, 0/255, 1.0))
If you will have any questions, don’t hesitate to ask 