I’m trying to make a script that uses the cursor script from the Defold Input library and using the example given in Github as a basis, however, the message.id, and message.group always comes back as nil and I don’t know why.
Settings for the collision object in each of the game objects I want to be clickable and draggable.
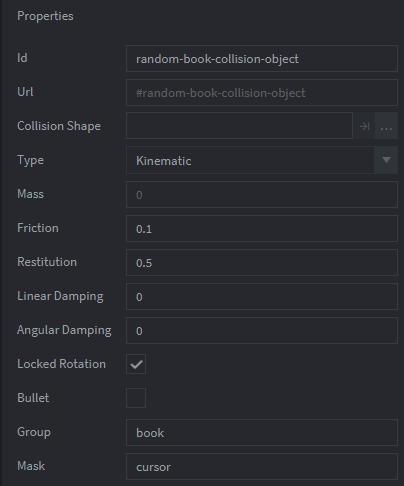
Code in controller.script
local cursor = require "in.cursor"
function init(self)
if not go.get("#cursor", "acquire_input_focus") then
self.forward_input = true
msg.post(".", "acquire_input_focus")
end
end
function on_input(self, action_id, action)
if self.forward_input then
msg.post("#cursor", "input", { action_id = action_id, action = action })
end
end
function on_message(self, message_id, message, sender)
if message_id == cursor.OVER then
print("Cursor over", message.id, message.group, message.x, message.y)
elseif message_id == cursor.OUT then
print("Cursor out", message.id, message.group, message.x, message.y)
elseif message_id == cursor.PRESSED then
print("Pressed", message.id, message.group, message.x, message.y)
elseif message_id == cursor.RELEASED then
print("Released", message.id, message.group, message.x, message.y)
elseif message_id == cursor.CLICKED then
print("Clicked", message.id, message.group, message.x, message.y)
if message.group == hash("computer") then
msg.post("/computer#computer", "acquire_input_focus")
end
elseif message_id == cursor.DRAG_START then
print("Drag started", message.id, message.group, message.x, message.y)
elseif message_id == cursor.DRAG_END then
print("Drag ended", message.id, message.group, message.x, message.y)
elseif message_id == cursor.DRAG then
print("Drag", message.id, message.group, message.x, message.y, message.dx, message.dy)
end
end
book.script
local cursor = require "in.cursor"
function on_message(self, message_id, message, sender)
if message_id == cursor.OVER then
self.over_pos = go.get_position()
go.set_position(vmath.vector3(self.over_pos.x, self.over_pos.y, 1))
go.animate("#sprite", "tint.w", go.PLAYBACK_ONCE_FORWARD, 1.5, go.EASING_OUTQUAD, 0.1)
elseif message_id == cursor.OUT then
local pos = go.get_position()
go.set_position(vmath.vector3(pos.x, pos.y, self.over_pos.z))
go.animate("#sprite", "tint.w", go.PLAYBACK_ONCE_FORWARD, 1, go.EASING_OUTQUAD, 0.1)
end
end
If I click on the computer, it should have the input focus, but if I click somewhere else or another object like the book, if I drag the book it should move. Only it doesn’t for some reason, I’m slightly confused by how the groups and masks on collision objects work. I’m reading Collision messages in Defold so I get where message.group is coming from, but if I just want the behavior to be specific to only a game object, how does other_id
work. Like it states to get the instance’s id, but how would you do that, isn’t every different instance going to have a different id?
Thanks to anyone who answers.