No problem, that’s my bad, I’ll just post all of what I have.
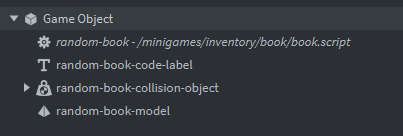
book.script
of book.go
function init(self)
generate_book_appearance()
generate_book_code()
end
local function generate_book_code()
self.book_code = ""
local charset = {} do
-- Ký tự [0-9a-zA-Z] dưới dạng mã ASCII: https://www.rapidtables.com/code/text/ascii-table.html
for c = 48, 57 do table.insert(charset, string.char(c)) end
for c = 65, 90 do table.insert(charset, string.char(c)) end
for c = 97, 122 do table.insert(charset, string.char(c)) end
end
-- [0-9a-zA-Z]{4}-[0-9a-zA-Z]{4}-[0-9a-zA-Z]{4}-[0-9a-zA-Z]{4}
while (string.len(self.book_code) <= 19) do
if (#self.book_code % 5 == 0) then
self.book_code = self.book_code .."-"
else
self.book_code = self.book_code .. charset[math.random(1, #charset)]
end
end
label.set_text("#random_book_code_label", self.book_code)
msg.post("/computer#computer", "actual_book_code", self.book_code)
end
local function generate_book_appearance()
go.set("#random-book-model", "tint", vmath.vector4(1,0,0,1))
end
which is being spawned via random_book_factory
from insert-book-code.script
.
local function delete_book(self)
if self.book_id then
go.set(self.book_id, "position.x", -1.0)
go.animate(self.book_id, "position.x", go.PLAYBACK_ONCE_FORWARD, 100, go.EASING_INQUART, 2)
sound.play(self.book_id.."#sliding-sound")
go.delete(self.book_id)
self.book_id = nil
end
end
local function create_book(self)
-- attempt to call global 'delete_book' (a nil value)
delete_book(self)
self.book_id = factory.create("#random_book_factory", vmath.vector3(-1.0, 0, 0))
go.set(self.book_id, "position.x", 0.0)
go.animate(self.book_id, "position.x", go.PLAYBACK_ONCE_FORWARD, 100, go.EASING_INQUART, 2)
sound.play(self.book_id.."#sliding-sound")
end
function init(self)
msg.post("/computer#computer", "acquire_input_focus")
create_book(self)
timer.delay(8, false, function()
msg.post("main:/main", "game_over")
end)
end
function on_message(self, message_id, message, sender)
-- still trying to figure how to delete book then respawn it again after the code's accepted
if message_id == hash("delete_book") then
delete_book()
end
end
then inside another game object in the same collection, computer.go
, I have a script called computer.script
. Basically I’m trying to make a ‘check in’ system. A random book spawns, the player types in the computer and has to match the code on it, then if it matches, it destroys the book and the book gets created again until the timer runs out and the collection unloads.
local actual_book_code = ""
function init(self)
self.message = ""
end
function on_input(self, action_id, action)
if action_id == hash("type") then
msg.post("/computer_sound#computer-sound", "play_keyboard")
self.message = self.message .. action.text
label.set_text("#code_input_box", self.message)
elseif action_id == hash("backspace") and action.repeated then
msg.post("/computer_sound#computer-sound", "play_keyboard")
local l = string.len(self.message)
self.message = string.sub(self.message, 0, l-1)
label.set_text("#code_input_box", self.message)
elseif action_id==hash("enter") then
msg.post("/computer_sound#computer-sound", "play_keyboard")
if self.message == actual_book_code then
msg.post("/minigame_go#insert-book_code", "delete_book")
end
end
end
function on_message(self, message_id, message, sender)
if message_id == hash("actual_book_code") then
actual_book_code = message.book_code
end
end
I was reading Game object API documentation at go.animate and seeing property: id of the property to animate
so I thought I could pass in the position, and I remember seeing an example too. I’m probably making a lot of errors in the code and am trying to figure out how to decouple.