Hi!
I’m animating the X and Y positions of several gui nodes (labels) using gui.animate. They are slowly going out of sync (it’s a looped anim)
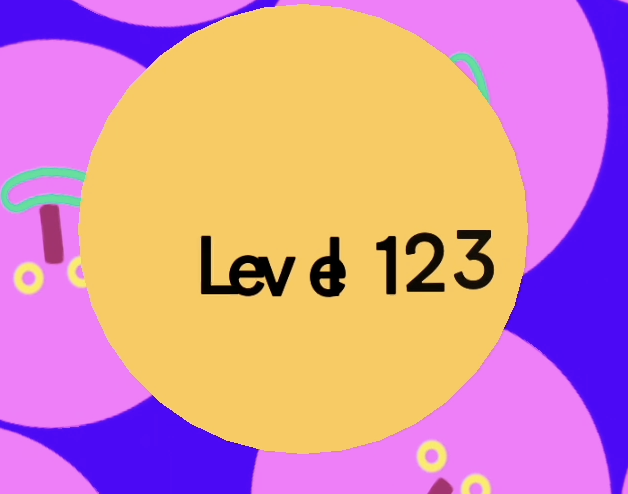
local number = 8
local xdis = 200
local ydis = 30
local spread = 0.1
local time = 4
local table = {
10,9,8,8,5,
10,10,10
}
for i = 1,number do
local node = gui.get_node("text"..i)
gui.set_position(node, vmath.vector3(-xdis, ydis, 0))
local accum = 0
for n = 1, i do
accum = accum + (table[n]/100)
end
gui.set_color(node, vmath.vector4(0,0,0,0))
gui.animate(node, "color.w", 1, gui.EASING_INOUTQUAD, time, accum+(time/4), nil, gui.PLAYBACK_LOOP_PINGPONG)
gui.animate(node, "position.x", xdis, gui.EASING_INOUTSINE, time, accum, nil, gui.PLAYBACK_LOOP_PINGPONG)
gui.animate(node, "position.y", -ydis, gui.EASING_INOUTSINE, time, accum+(time/4), nil, gui.PLAYBACK_LOOP_PINGPONG)
end
timer.delay(0.2, true, function(self)
for i = 1,number do
local node = gui.get_node("text"..i)
local pos = gui.get_position(node)
if pos.y > 0 then
gui.set_layer(node, "behind")
else
gui.set_layer(node, "infront")
end
end
end)
Any help? Would I get any benefit from using a timer and repeating gui.animate instead of using the Pingpong loop?
thanks.