Ok, first version would be something like this:
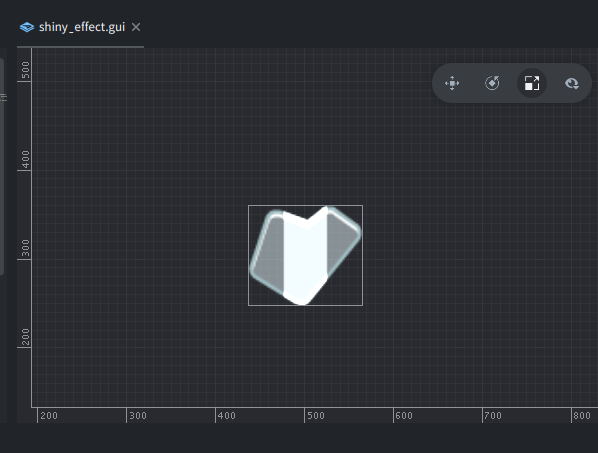
Fragment program for this is very simple:
varying mediump vec2 var_texcoord0;
varying lowp vec4 var_color;
uniform lowp sampler2D texture_sampler;
uniform lowp vec4 tint;
uniform mediump vec4 stripe_data; // (x - position, y - width, z - sharpness, w - angle)
void main()
{
lowp vec4 tex = texture2D(texture_sampler, var_texcoord0.xy);
// Calculate the distance from the stripe center
mediump float dist_from_stripe = abs(var_texcoord0.x - stripe_data.x);
// Compute the stripe intensity with sharpness
mediump float stripe = 1.0 - smoothstep(
stripe_data.y * (1.0 - stripe_data.z), // Start of transition
stripe_data.y, // End of transition
dist_from_stripe
);
// Create the stripe effect as a highlight
lowp vec4 highlight = vec4(1.0) * stripe;
// Add stripe highlight to non-transparent pixels
gl_FragColor = tex * var_color + highlight * tex.w;
}
Adding rotation complicates it a bit, but all you need is rotated_coords
addition:
// Rotate texture coordinates
mediump float cos_angle = cos(stripe_data.w);
mediump float sin_angle = sin(stripe_data.w);
mediump vec2 rotated_coords = vec2(
var_texcoord0.x * cos_angle - var_texcoord0.y * sin_angle,
var_texcoord0.x * sin_angle + var_texcoord0.y * cos_angle
);
And use rotated_coords
in dist_from_stripe
instead.
You can animate it with gui.set()
in a .gui_script:
function init(self)
self.box_node = gui.get_node("box")
self.position_x = 0
end
function update(self, dt)
self.position_x = self.position_x + 0.01
if self.position_x > 1.5 then self.position_x = -0.5 end
gui.set(self.box_node, "stripe_data", vmath.vector4(self.position_x, 0.2, 0.2, 0))
end
Effect:
Project (let it be also my entry to Defold Community Challenge
):